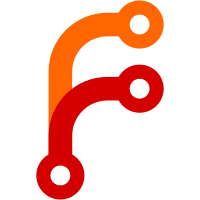
This adds RHEL8's `/usr/libexec/platform-python` to Spack's list of preferred pythons. It will only be used if no other `python` is available in the `PATH`. We have been testing with this python for a while now, and it seems to do all that we need. If Spack one day isn't able to work with it, we'll take it out, but for now it is useful to allow Spack to be used on RHEL8 without a dedicated `python` installation.
77 lines
2.6 KiB
Python
Executable file
77 lines
2.6 KiB
Python
Executable file
#!/bin/sh
|
|
# -*- python -*-
|
|
#
|
|
# Copyright 2013-2021 Lawrence Livermore National Security, LLC and other
|
|
# Spack Project Developers. See the top-level COPYRIGHT file for details.
|
|
#
|
|
# SPDX-License-Identifier: (Apache-2.0 OR MIT)
|
|
|
|
# This file is bilingual. The following shell code finds our preferred python.
|
|
# Following line is a shell no-op, and starts a multi-line Python comment.
|
|
# See https://stackoverflow.com/a/47886254
|
|
""":"
|
|
# prefer SPACK_PYTHON environment variable, python3, python, then python2
|
|
SPACK_PREFERRED_PYTHONS="python3 python python2 /usr/libexec/platform-python"
|
|
for cmd in "${SPACK_PYTHON:-}" ${SPACK_PREFERRED_PYTHONS}; do
|
|
if command -v > /dev/null "$cmd"; then
|
|
export SPACK_PYTHON="$(command -v "$cmd")"
|
|
exec "${SPACK_PYTHON}" "$0" "$@"
|
|
fi
|
|
done
|
|
|
|
echo "==> Error: spack could not find a python interpreter!" >&2
|
|
exit 1
|
|
":"""
|
|
# Line above is a shell no-op, and ends a python multi-line comment.
|
|
# The code above runs this file with our preferred python interpreter.
|
|
|
|
from __future__ import print_function
|
|
|
|
import os
|
|
import sys
|
|
|
|
min_python3 = (3, 5)
|
|
|
|
if sys.version_info[:2] < (2, 6) or (
|
|
sys.version_info[:2] >= (3, 0) and sys.version_info[:2] < min_python3
|
|
):
|
|
v_info = sys.version_info[:3]
|
|
msg = "Spack requires Python 2.6, 2.7 or %d.%d or higher " % min_python3
|
|
msg += "You are running spack with Python %d.%d.%d." % v_info
|
|
sys.exit(msg)
|
|
|
|
# Find spack's location and its prefix.
|
|
spack_file = os.path.realpath(os.path.expanduser(__file__))
|
|
spack_prefix = os.path.dirname(os.path.dirname(spack_file))
|
|
|
|
# Allow spack libs to be imported in our scripts
|
|
spack_lib_path = os.path.join(spack_prefix, "lib", "spack")
|
|
sys.path.insert(0, spack_lib_path)
|
|
|
|
# Add external libs
|
|
spack_external_libs = os.path.join(spack_lib_path, "external")
|
|
|
|
if sys.version_info[:2] <= (2, 7):
|
|
sys.path.insert(0, os.path.join(spack_external_libs, "py2"))
|
|
if sys.version_info[:2] == (2, 6):
|
|
sys.path.insert(0, os.path.join(spack_external_libs, "py26"))
|
|
|
|
sys.path.insert(0, spack_external_libs)
|
|
|
|
# Here we delete ruamel.yaml in case it has been already imported from site
|
|
# (see #9206 for a broader description of the issue).
|
|
#
|
|
# Briefly: ruamel.yaml produces a .pth file when installed with pip that
|
|
# makes the site installed package the preferred one, even though sys.path
|
|
# is modified to point to another version of ruamel.yaml.
|
|
if "ruamel.yaml" in sys.modules:
|
|
del sys.modules["ruamel.yaml"]
|
|
|
|
if "ruamel" in sys.modules:
|
|
del sys.modules["ruamel"]
|
|
|
|
import spack.main # noqa
|
|
|
|
# Once we've set up the system path, run the spack main method
|
|
if __name__ == "__main__":
|
|
sys.exit(spack.main.main())
|