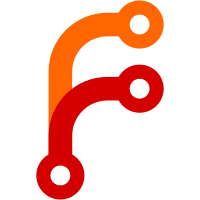
- in FOAM library updated containers backported PackedBoolList, hashedWordList, nullObject, wordRe, backported functions to backported int32 support backported tableReaders backported Function1, TimeFunction1 backported dynamicCode (for codedBCs, ...) -- needs to be mapped out advanced error macros (FatalIOErrorInFunction, ...) -- needs to be mapped out backported IOobject::MUST_READ_IF_MODIFIED and added IOobject::READ_IF_PRESENT_IF_MODIFIED (only in FO) - in postProcessing backported IO FOs (partialWrite, removeRegisteredObject, writeDictionary, writeRegisteredObject) backported field FOs (fieldCoordinateSystemTransform, fieldValues, nearWallFields, processorField, readFields, regionSizeDistribution, streamLine, wallBoundedStreamLine) backported fvTools FOs (calcFvcDiv, calcFvcGrad, calcMag) backported jobControl FOs (abortCalculation) backported utilities FOs (ourantNo, Lambda2, Peclet, Q, codedFunctionObject, pressureTools, residuals, scalarTransport, setTimeStep, timeActivatedFileUpdate, turbulenceFields, vorticity, wallShearStress)
182 lines
3.3 KiB
C++
182 lines
3.3 KiB
C++
Info<< "Reading field alpha\n" << endl;
|
|
volScalarField alpha
|
|
(
|
|
IOobject
|
|
(
|
|
"alpha",
|
|
runTime.timeName(),
|
|
mesh,
|
|
IOobject::MUST_READ,
|
|
IOobject::AUTO_WRITE
|
|
),
|
|
mesh
|
|
);
|
|
|
|
volScalarField beta
|
|
(
|
|
IOobject
|
|
(
|
|
"beta",
|
|
runTime.timeName(),
|
|
mesh,
|
|
IOobject::NO_READ,
|
|
IOobject::NO_WRITE
|
|
),
|
|
scalar(1) - alpha
|
|
//,alpha.boundaryField().types()
|
|
);
|
|
|
|
Info<< "Reading field p\n" << endl;
|
|
volScalarField p
|
|
(
|
|
IOobject
|
|
(
|
|
"p",
|
|
runTime.timeName(),
|
|
mesh,
|
|
IOobject::MUST_READ,
|
|
IOobject::AUTO_WRITE
|
|
),
|
|
mesh
|
|
);
|
|
|
|
Info<< "Reading field Ua\n" << endl;
|
|
volVectorField Ua
|
|
(
|
|
IOobject
|
|
(
|
|
"Ua",
|
|
runTime.timeName(),
|
|
mesh,
|
|
IOobject::MUST_READ,
|
|
IOobject::AUTO_WRITE
|
|
),
|
|
mesh
|
|
);
|
|
|
|
Info<< "Reading field Ub\n" << endl;
|
|
volVectorField Ub
|
|
(
|
|
IOobject
|
|
(
|
|
"Ub",
|
|
runTime.timeName(),
|
|
mesh,
|
|
IOobject::MUST_READ,
|
|
IOobject::AUTO_WRITE
|
|
),
|
|
mesh
|
|
);
|
|
|
|
volVectorField U
|
|
(
|
|
IOobject
|
|
(
|
|
"U",
|
|
runTime.timeName(),
|
|
mesh,
|
|
IOobject::NO_READ,
|
|
IOobject::AUTO_WRITE
|
|
),
|
|
alpha*Ua + beta*Ub
|
|
);
|
|
|
|
|
|
Info<< "Reading transportProperties\n" << endl;
|
|
|
|
IOdictionary transportProperties
|
|
(
|
|
IOobject
|
|
(
|
|
"transportProperties",
|
|
runTime.constant(),
|
|
mesh,
|
|
IOobject::MUST_READ_IF_MODIFIED,
|
|
IOobject::NO_WRITE
|
|
)
|
|
);
|
|
|
|
dimensionedScalar rhoa
|
|
(
|
|
transportProperties.lookup("rhoa")
|
|
);
|
|
|
|
dimensionedScalar rhob
|
|
(
|
|
transportProperties.lookup("rhob")
|
|
);
|
|
|
|
dimensionedScalar nua
|
|
(
|
|
transportProperties.lookup("nua")
|
|
);
|
|
|
|
dimensionedScalar nub
|
|
(
|
|
transportProperties.lookup("nub")
|
|
);
|
|
|
|
dimensionedScalar da
|
|
(
|
|
transportProperties.lookup("da")
|
|
);
|
|
|
|
dimensionedScalar db
|
|
(
|
|
transportProperties.lookup("db")
|
|
);
|
|
|
|
dimensionedScalar Cvm
|
|
(
|
|
transportProperties.lookup("Cvm")
|
|
);
|
|
|
|
dimensionedScalar Cl
|
|
(
|
|
transportProperties.lookup("Cl")
|
|
);
|
|
|
|
dimensionedScalar Ct
|
|
(
|
|
transportProperties.lookup("Ct")
|
|
);
|
|
|
|
# include "createPhia.H"
|
|
# include "createPhib.H"
|
|
|
|
surfaceScalarField phi
|
|
(
|
|
IOobject
|
|
(
|
|
"phi",
|
|
runTime.timeName(),
|
|
mesh
|
|
),
|
|
fvc::interpolate(alpha)*phia
|
|
+ fvc::interpolate(beta)*phib
|
|
);
|
|
|
|
|
|
# include "createRASTurbulence.H"
|
|
|
|
Info<< "Calculating field DDtUa and DDtUb\n" << endl;
|
|
|
|
volVectorField DDtUa =
|
|
fvc::ddt(Ua)
|
|
+ fvc::div(phia, Ua)
|
|
- fvc::div(phia)*Ua;
|
|
|
|
volVectorField DDtUb =
|
|
fvc::ddt(Ub)
|
|
+ fvc::div(phib, Ub)
|
|
- fvc::div(phib)*Ub;
|
|
|
|
|
|
Info<< "Calculating field g.h\n" << endl;
|
|
volScalarField gh("gh", g & mesh.C());
|
|
|
|
|
|
label pRefCell = 0;
|
|
scalar pRefValue = 0.0;
|
|
setRefCell(p, piso.dict(), pRefCell, pRefValue);
|
|
mesh.schemesDict().setFluxRequired(p.name());
|