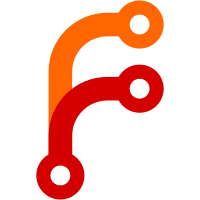
- in FOAM library updated containers backported PackedBoolList, hashedWordList, nullObject, wordRe, backported functions to backported int32 support backported tableReaders backported Function1, TimeFunction1 backported dynamicCode (for codedBCs, ...) -- needs to be mapped out advanced error macros (FatalIOErrorInFunction, ...) -- needs to be mapped out backported IOobject::MUST_READ_IF_MODIFIED and added IOobject::READ_IF_PRESENT_IF_MODIFIED (only in FO) - in postProcessing backported IO FOs (partialWrite, removeRegisteredObject, writeDictionary, writeRegisteredObject) backported field FOs (fieldCoordinateSystemTransform, fieldValues, nearWallFields, processorField, readFields, regionSizeDistribution, streamLine, wallBoundedStreamLine) backported fvTools FOs (calcFvcDiv, calcFvcGrad, calcMag) backported jobControl FOs (abortCalculation) backported utilities FOs (ourantNo, Lambda2, Peclet, Q, codedFunctionObject, pressureTools, residuals, scalarTransport, setTimeStep, timeActivatedFileUpdate, turbulenceFields, vorticity, wallShearStress)
176 lines
4.6 KiB
C++
176 lines
4.6 KiB
C++
/*---------------------------------------------------------------------------*\
|
|
========= |
|
|
\\ / F ield | foam-extend: Open Source CFD
|
|
\\ / O peration | Version: 4.0
|
|
\\ / A nd | Web: http://www.foam-extend.org
|
|
\\/ M anipulation | For copyright notice see file Copyright
|
|
-------------------------------------------------------------------------------
|
|
License
|
|
This file is part of foam-extend.
|
|
|
|
foam-extend is free software: you can redistribute it and/or modify it
|
|
under the terms of the GNU General Public License as published by the
|
|
Free Software Foundation, either version 3 of the License, or (at your
|
|
option) any later version.
|
|
|
|
foam-extend is distributed in the hope that it will be useful, but
|
|
WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with foam-extend. If not, see <http://www.gnu.org/licenses/>.
|
|
|
|
Class
|
|
Foam::FUNCTIONOBJECT
|
|
|
|
Group
|
|
|
|
Description
|
|
This function object...
|
|
|
|
Example of function object specification:
|
|
\verbatim
|
|
FUNCTIONOBJECT1
|
|
{
|
|
type FUNCTIONOBJECT;
|
|
functionObjectLibs ("libFUNCTIONOBJECTFunctionObject.so");
|
|
...
|
|
wordData someWord;
|
|
scalarData 1.0;
|
|
labelData 1;
|
|
}
|
|
\endverbatim
|
|
|
|
\heading Function object usage
|
|
\table
|
|
Property | Description | Required | Default value
|
|
type | type name: FUNCTIONOBJECT | yes |
|
|
wordData | some word option... | no | defaultWord
|
|
scalarData | some scalar value... | yes |
|
|
labelData | some label value... | yes |
|
|
\endtable
|
|
|
|
SourceFiles
|
|
FUNCTIONOBJECT.C
|
|
IOFUNCTIONOBJECT.H
|
|
|
|
\*---------------------------------------------------------------------------*/
|
|
|
|
#ifndef FUNCTIONOBJECT_H
|
|
#define FUNCTIONOBJECT_H
|
|
|
|
#include "runTimeSelectionTables.H"
|
|
|
|
// * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * //
|
|
|
|
namespace Foam
|
|
{
|
|
|
|
// Forward declaration of classes
|
|
class objectRegistry;
|
|
class dictionary;
|
|
class polyMesh;
|
|
class mapPolyMesh;
|
|
|
|
/*---------------------------------------------------------------------------*\
|
|
Class FUNCTIONOBJECT Declaration
|
|
\*---------------------------------------------------------------------------*/
|
|
|
|
class FUNCTIONOBJECT
|
|
{
|
|
// Private data
|
|
|
|
//- Name of this set of FUNCTIONOBJECT
|
|
word name_;
|
|
|
|
//- Refererence to Db
|
|
const objectRegistry& obr_;
|
|
|
|
// Read from dictionary
|
|
|
|
//- word
|
|
word wordData_;
|
|
|
|
//- scalar
|
|
scalar scalarData_;
|
|
|
|
//- label
|
|
label labelData_;
|
|
|
|
|
|
// Private Member Functions
|
|
|
|
|
|
//- Disallow default bitwise copy construct
|
|
FUNCTIONOBJECT(const FUNCTIONOBJECT&);
|
|
|
|
//- Disallow default bitwise assignment
|
|
void operator=(const FUNCTIONOBJECT&);
|
|
|
|
|
|
public:
|
|
|
|
//- Runtime type information
|
|
TypeName("FUNCTIONOBJECT");
|
|
|
|
|
|
// Constructors
|
|
|
|
//- Construct for given objectRegistry and dictionary.
|
|
// Allow the possibility to load fields from files
|
|
FUNCTIONOBJECT
|
|
(
|
|
const word& name,
|
|
const objectRegistry&,
|
|
const dictionary&,
|
|
const bool loadFromFiles = false
|
|
);
|
|
|
|
|
|
//- Destructor
|
|
virtual ~FUNCTIONOBJECT();
|
|
|
|
|
|
// Member Functions
|
|
|
|
//- Return name of the FUNCTIONOBJECT
|
|
virtual const word& name() const
|
|
{
|
|
return name_;
|
|
}
|
|
|
|
//- Read the FUNCTIONOBJECT data
|
|
virtual void read(const dictionary&);
|
|
|
|
//- Execute, currently does nothing
|
|
virtual void execute();
|
|
|
|
//- Execute at the final time-loop, currently does nothing
|
|
virtual void end();
|
|
|
|
//- Called when time was set at the end of the Time::operator++
|
|
virtual void timeSet();
|
|
|
|
//- Write the FUNCTIONOBJECT
|
|
virtual void write();
|
|
|
|
//- Update for changes of mesh
|
|
virtual void updateMesh(const mapPolyMesh&)
|
|
{}
|
|
|
|
//- Update for changes of mesh
|
|
virtual void movePoints(const polyMesh&)
|
|
{}
|
|
};
|
|
|
|
|
|
// * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * //
|
|
|
|
} // End namespace Foam
|
|
|
|
// * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * * //
|
|
|
|
#endif
|
|
|
|
// ************************************************************************* //
|